by kirupa |
7 March
2007
In the
previous page you learned how to detect individual key
presses. Another important thing you would want to do is
detect combination key presses, and you just saw the code
for doing that. Let's pick up from there and look at the implementation for Ctrl + S in greater
detail:
- // Ctrl + S
- if
((Keyboard.Modifiers
==
ModifierKeys.Control)
&& (e.Key
== Key.S))
- {
- ProcessSaveCommand();
- }
To check if the Ctrl key has been pressed, I cannot
access the Key enum and
access the Ctrl key like I would any other key from the
keyboard. This is the approach you used earlier to detect
when the K key was pressed.
The key approach doesn't work for another reason because
your commonly used Alt, Ctrl,
Shift, and Windows keys
can't be accessed from the Key enum at all. Instead, those
four keys can only be accessed using the
ModifierKeys enum and
checking whether Keyboard.Modifiers
is equal to that key. That's what is done in the first
condition of the if statement for the Ctrl key:
- Keyboard.Modifiers
==
ModifierKeys.Control
Learning to use ModifierKeys
and setting it equal to
Keyboard.Modifiers is really the tricky part of
implementing key combinations. For the remaining keys, you
can access them directly by checking if the key passed in by
your event argument e is
equal to the key on the keyboard you are checking. That can
be seen in the second part of the if statement which takes
care of your normal (non-modifier) key S:
- (Keyboard.Modifiers
==
ModifierKeys.Control)
&& (e.Key
== Key.S)
One final thing to note is that you use ampersands to see
if your modifier key is pressed along with your key. Think
of key combinations as checking whether two inequalities are
equal. In the above code, I check if the Control (Ctrl)
modifier key has been pressed and whether the second key
that has been pressed is the S key. When both conditions are
true, then your application recognizes your key combination.
If you noticed, you added the above code to the event
handler mapping KeyUp. That
wasn't done arbitrarily, for there is a real good reason why
KeyUp was used instead of
KeyDown. When you are using
key combinations, for example Ctrl + S, remember that you
are actually pressing the keyboard buttons Ctrl and S. If
you map those events to KeyDown you will find that not only
is your application interpreting the Ctrl + S, it is sending
those keys for display on the screen. You probably do not
want that.
The result is something similar to what you see in the
following image. Notice that after pressing Ctrl + S, the s
character also displays in the textbox:
[ notice that the key
combination also results in the pressed letter being shown ]
You can avoid having the above problem by having your key
combination code located in an event handler linked to
KeyUp.
Just a final word before we wrap up. If you have a question and/or want to be part of a friendly, collaborative community of over 220k other developers like yourself, post on the forums for a quick response!
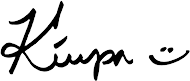
|