by kirupa |
5 January
2007
In the
previous page you created a class and learned about
constructors. We will pick up from where we left off and
learn how to add more functionality to our class using
methods.
Right now, our Planet class only contains fields and a
constructor. Constructors are great for simply creating your object
and initializing fields, but beyond that, they cannot help
you. In order to have your class do more interesting things,
you will need to use what are called methods.
In a nutshell, a method is a block of code that does
something specific. It has a name and various tags
(properties) that describe its behavior. More importantly,
methods also have the ability to return data back to whoever
called it. For example, you probably have been using a
method called Main to test your programs:
- static
void
Main(string[]
args)
- {
- Planet
earth
=
new Planet(6378,
9.81,
"Earth");
-
- Console.WriteLine("Earth's
radius is: " +
earth.radius);
- }
To best describe the individual parts that make up a
method, let's look at the following image:
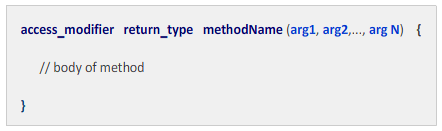
Your method declaration follows the template shown in the
above image. The following list explains what each part
means:
Access modifiers define how easily accessible your
method will be, and this will be very important when I
explain inheritance. The access modifiers we learned so
far are public and
private, and the other modifiers,
which I will cover on a later date, are
protected, static,
internal, readonly,
volatile, new,
and protected internal.
The type of an object is basically the class it belongs
to. The return-type is then the type of the data you are
returning when the method is called. If you set your
return type to void, that means that you are not sending
any data back. We'll see an example of this in use
shortly.
This is pretty easy! This is just the name you give your
method.
Your method can take in values much like how our
constructor took in values to assign values to our
fields.
Your method's code would go here.
Now that you have a basic idea of what goes into a
method, let's look at the first type of method I will cover,
Instance Methods.
Instance methods are methods you
call from a object. The best way to explain it is via an
example. For the past few pages, we have tried to get
data from our
name field to display when accessed via our object.
Let's use a method to do that right now.
Add the following non-grayed out code to your Planet
class:
- class Planet
- {
- public int radius;
- public double gravity;
- private string name;
-
- // A Constructor
- public Planet(int r, double g, string n)
- {
- radius = r;
- gravity = g;
- name = n;
- }
-
- //Return the object's
name!
- public
string
GetName()
- {
- return
name;
- }
- }
More specifically, the code you added is shown below:
- //Return the object's name!
- public
string
GetName()
- {
- return
name;
- }
Let's dissect our GetName() method. Notice that its
return type is string, and in the body of our method, we
return the name field which is also of type string. The
return type of your method must match the return type of the
value being returned. Finally, notice that the access
modifier of our method is public. Guess what that means?
You'll find out when you add the following code to your
Main method:
- static
void
Main(string[] args)
- {
- Planet
earth
=
new Planet(6378, 9.81,
"Earth");
-
- Console.WriteLine("Earth's
radius is: " +
earth.radius);
- Console.WriteLine("Earth's
name is: " +
earth.GetName());
- }
Notice that when you run your application by pressing
Ctrl + F5, you will see not only the radius being being
displayed, but the name is also displayed:
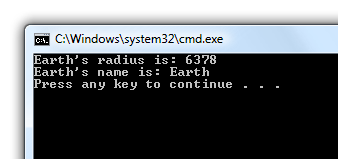
[ your output window with the radius and name information
displayed ]
The reason the name is displayed is because while the
name field is private, the method that returns the value is
public. Our goal was to hide the name field, but not to hide
the GetName() method. Since GetName() is within our Planet
class definition, returning the private name field is
allowed.
Onwards to the
next
page!
|